Welcome to another one of my interesting findings, where I explore some stuff for fun and profit just fun A while back I was solving AOC and for a problem (day 13), I had this sort of clever idea of using eval to skip writing a if else ladder. The input file had the following structure Starting items: 91, 65 Operation: new = old * 13 Test: divisible by 5 If true: throw to monkey 7 If false: throw to monkey 4 ...
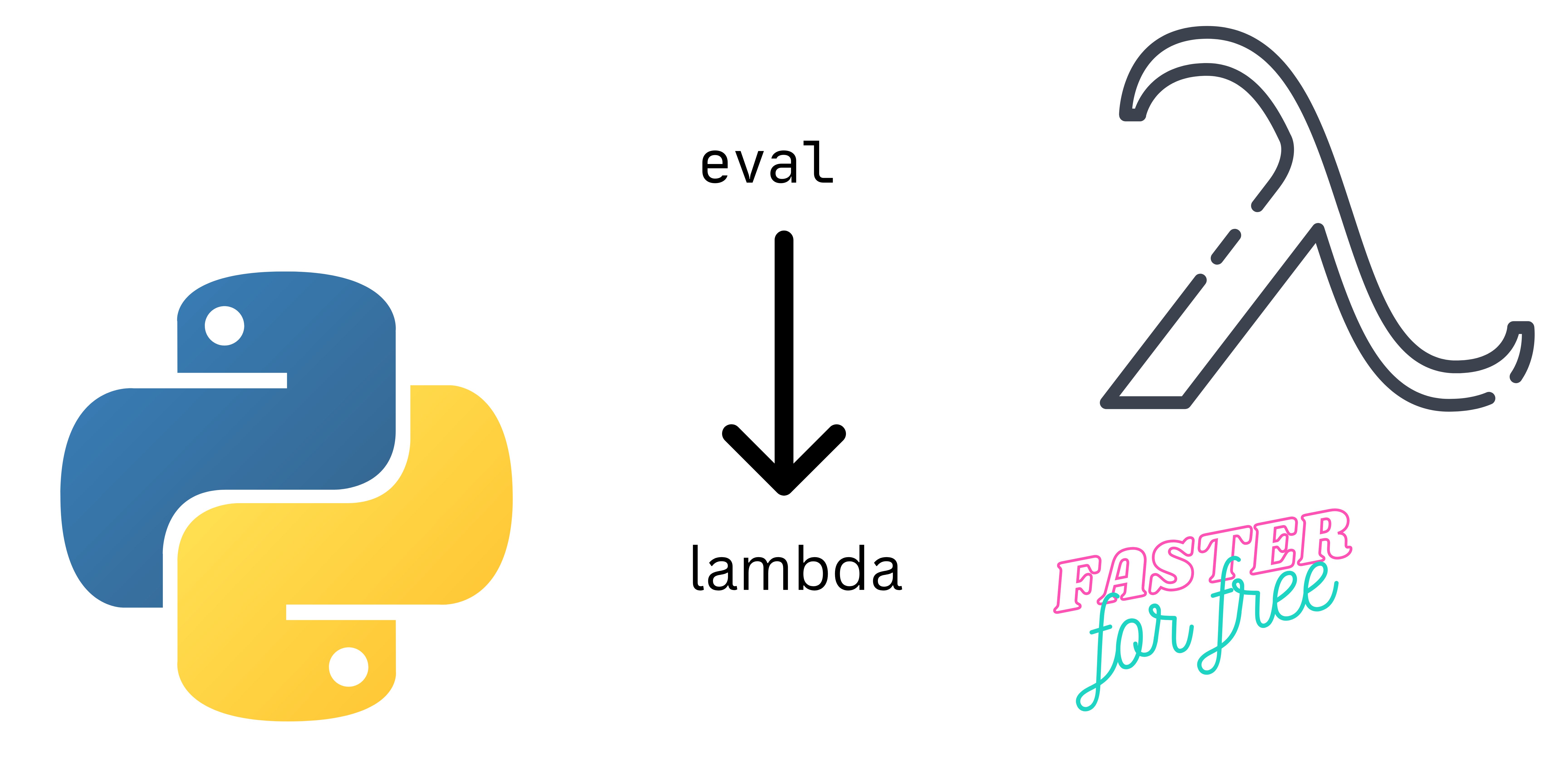